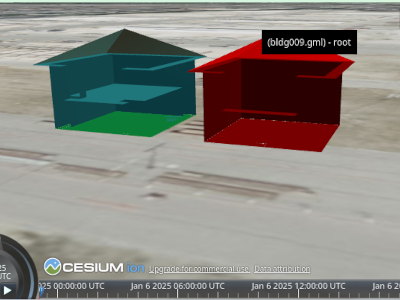
Highlight and show an attribute as label on map by hover in Cesium
Personal
JavaScript
CesiumJS
- sets a nameOverlay HTML object to show attributes
- assign a custom style to highlight a selected instance of 3DTiles
- checks available attributes of an instance
sets a nameOverlay HTML object to show attributes
assign a custom style to highlight a selected instance of 3DTiles
checks available attributes of an instance
Description :
Simple Expression :
// HTML overlay for showing feature name on mouseover
const nameOverlay = document.createElement('div');
// ...
// Pick a new feature
let pickedFeature = viewer.scene.pick(movement.endPosition);
// ...
// Highlight the feature if it's not already selected.
if (pickedFeature !== selected.feature) {
highlighted.feature = pickedFeature;
Cesium.Color.clone(pickedFeature.color, highlighted.originalColor);
pickedFeature.color = Cesium.Color.RED;
}
Example :
// Grant CesiumJS access to your ion assets
Cesium.Ion.defaultAccessToken = "XXX";
const viewer = new Cesium.Viewer("cesiumContainer");
viewer.scene.globe.depthTestAgainstTerrain = true;
try {
const default_style = new Cesium.Cesium3DTileStyle({
"show" : "true",
"color" : "color('#40E0D0')"
});
const tileset = await Cesium.Cesium3DTileset.fromIonAssetId(2734145);
viewer.scene.primitives.add(tileset);
await viewer.zoomTo(tileset);
tileset.style = default_style;
} catch (error) {
console.log(error);
}
// HTML overlay for showing feature name on mouseover
const nameOverlay = document.createElement('div');
viewer.container.appendChild(nameOverlay);
nameOverlay.className = 'backdrop';
nameOverlay.style.display = 'none';
nameOverlay.style.position = 'absolute';
nameOverlay.style.bottom = '0';
nameOverlay.style.left = '0';
nameOverlay.style['pointer-events'] = 'none';
nameOverlay.style.padding = '8px';
nameOverlay.style.backgroundColor = 'black';
// Information about the currently selected feature
const selected = {
feature: undefined,
originalColor: new Cesium.Color()
};
// An entity object which will hold info about the currently selected feature for infobox display
const selectedEntity = new Cesium.Entity();
const handler = new Cesium.ScreenSpaceEventHandler(viewer.canvas);
// Information about the currently highlighted feature
const highlighted = {
feature: undefined,
originalColor: new Cesium.Color()
};
// On mouse over, display all the properties for a feature in the console log.
handler.setInputAction(function onClick(movement) {
// If a feature was previously highlighted, undo the highlight
if (Cesium.defined(highlighted.feature)) {
highlighted.feature.color = highlighted.originalColor;
highlighted.feature = undefined;
}
const feature = viewer.scene.pick(movement.endPosition);
if (feature instanceof Cesium.Cesium3DTileFeature) {
// Pick a new feature
let pickedFeature = viewer.scene.pick(movement.endPosition);
if (!Cesium.defined(pickedFeature)) {
nameOverlay.style.display = 'none';
return;
}
try {
/*
const propertyIds = pickedFeature.getPropertyIds();
const length = propertyIds.length;
for (let i = 0; i < length; ++i) {
const propertyId = propertyIds[i];
console.log(`${propertyId}: ${pickedFeature.getProperty(propertyId)}`);
}*/
// A feature was picked, so show it's overlay content
nameOverlay.style.display = 'block';
nameOverlay.style.bottom = viewer.canvas.clientHeight - movement.endPosition.y + 'px';
nameOverlay.style.left = movement.endPosition.x + 'px';
// Following name setter would work for CityGML based 3DTiles
let name = '('+ pickedFeature.getProperty('FileName') + ') - ' + pickedFeature.getProperty('NodeName');
if (!Cesium.defined(name)) {
name = pickedFeature.getProperty('id');
}
//console.log(name);
nameOverlay.textContent = name;
}
catch (error) {
console.log(error);
}
// Highlight the feature if it's not already selected.
if (pickedFeature !== selected.feature) {
highlighted.feature = pickedFeature;
Cesium.Color.clone(pickedFeature.color, highlighted.originalColor);
pickedFeature.color = Cesium.Color.RED;
}
}
}, Cesium.ScreenSpaceEventType.MOUSE_MOVE);
2025 Jan